All Power Automate Text Functions (With Examples)
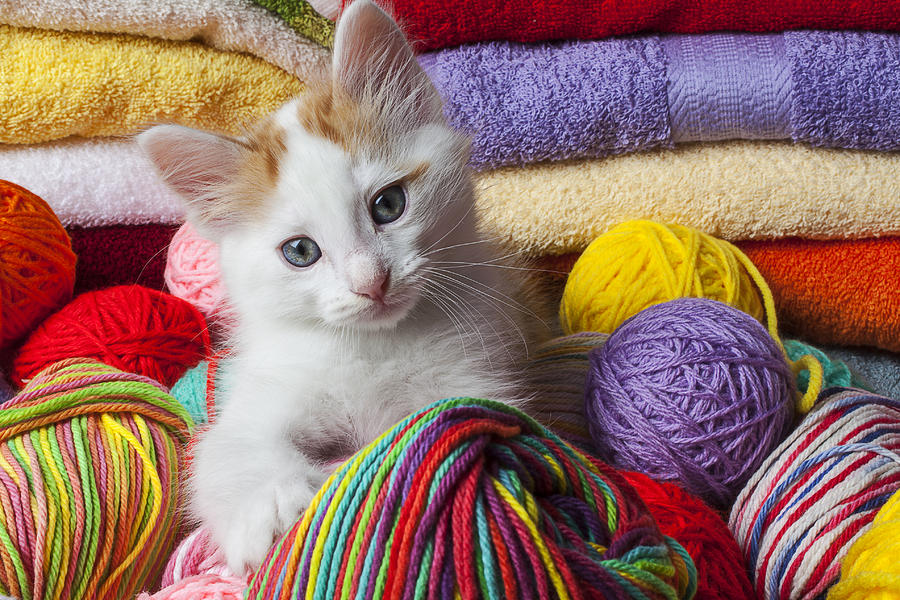
Power Automate text functions are frequently used when writing expressions. It is important to understand all of them and what they do. In this article I will list all of the Power Automate text functions and show examples of how to use them.
Table Of Contents:
• Chunk Function
• Concat Function
• EndsWith Function
• FormatNumber Function
• GUID Function
• IndexOf Function
• isFloat Function
• isInt Function
• LastIndexOf Function
• Length Function
• NthIndexOf Function
• Replace Function
• Slice Function
• Split Function
• StartsWith Function
• Substring Function
• ToLower Function
• ToUpper Function
• Trim Function
Chunk Function
Purpose
Split a text string into an array into chunks of a specified length
Syntax
chunk(collection, length)
Arguments
- collection – Required. The text string to split into chunks.
- length – Required. The length of the chunks as a whole number.
Example
Split a text string into 20 character chunks.
chunk('The quick brown fox jumps over the lazy dog', 20)
Result
[
"The quick brown fox ",
"jumps over the lazy ",
"dog"
]
Concat Function
Purpose
Join multiple text strings into a single text string
Syntax
concat(text1, text2, …)
Arguments
- text – Required. One or more text strings to be joined
Example
Join a folder path, filename and extension into a single text string.
concat('https://matthewdevaney.com/files/', 'samplefile', '.pdf')
Result
https://matthewdevaney.com/files/samplefile.pdf
EndsWith Function
Purpose
Determine whether a substring is found at the end of a text string and return a true/false value
Syntax
endsWith(text1, text2, …)
Arguments
- text – Required. Text string where the substring may be located
- searchText – Required. Substring to look for at the end of the text string
Example
Check if the file is an Excel file.
endsWith('Timesheet_MD_20231124.xlsx', '.xlsx')
Result
true
FormatNumber Function
Purpose
Change a number into a string with the specified format
Syntax
formatNumber(number, format, locale)
Arguments
- number – Required. The number to be formatted.
- format – Required. A text-based formatting code
- locale – Optional. Language code.
Example
Format a decimal number as currency.
formatNumber(1315.2,'$#,##0.00', 'en-us')
Result
$1,315.20
GUID Function
Purpose
Generates a random GUID.
Syntax
guid(format)
Arguments
- format- Optional. Default format is “D”. Can also be “B”, “N”, “P”, or “X”.
Example
Create a GUID value.
guid()
Result
536cebdf-7ec1-41c3-98bf-6a04efe7bfbe
IndexOf Function
Purpose
Find the starting position of a substring in a text string
Syntax
indexOf(text, searchText)
Arguments
- text – Required. Text string where the substring may be located.
- searchText – Required. Substring to look for the first occurrence of.
Example
Find where the position where the full name value starts based on the separator ‘: ‘.
indexOf('Full Name: Matthew Devaney', ': ')
Result
9
isFloat Function
Purpose
Determine whether a text string is of type float
Syntax
isFloat(string)
Arguments
- string – Required. Text string to be tested.
Example
Check whether the value “0.9723” is a float.
isFloat('0.9723')
Result
true
isInt Function
Purpose
Determine whether a text string is of type integer
Syntax
isInt(string)
Arguments
- string – Required. Text string to be tested.
Example
Check whether the value “10” is an integer.
isInt(10)
Result
true
LastIndexOf Function
Purpose
Find the last position of a substring in a text string
Syntax
lastIndexOf(text, searchText)
Arguments
- text – Required. Text string where the substring may be located.
- searchText – Required. Substring to look for the last occurrence of.
Example
Find the final comma in the comma-separated text string
lastIndexOf('01,3002,Accounts Receivable,50252.00',',')
Result
27
Length Function
Purpose
Returns the number of characters in a text string
Syntax
length(collection)
Arguments
- collection – Required. The text string to measure
Example
Determine how many characters are in the following sentence.
length('The quick brown fox jumps over the lazy dog')
Result
43
NthIndexOf Function
Purpose
Find the nth position of a substring in a text string
Syntax
nthIndexOf(text, searchText, occurence)
Arguments
- text – Required. Text string where the substring may be located.
- searchText – Required. Substring to look for the nth occurrence of.
- occurrence – Required. Nth number of the occurrence to look for.
Example
Find the 2nd comma in the comma-separated text string
nthIndexOf('01,3002,Accounts Receivable,50252.00',',',2)
Result
7
Replace Function
Purpose
Replace a substring of old text with another substring of new text.
Syntax
replace(text, oldText, newText)
Arguments
- text – Required. The text string containing the old text.
- oldText – Required. The old text to search for and replace.
- newText – Required. The new text to replace the old text with.
Example
Remove any commas from the text string by replacing them with a blank space.
replace('John, Bradley, Smith', ',', ' ')
Result
John Bradley Smith
Slice Function
Purpose
Extract a range of characters from the middle of a text string
Syntax
slice(text, startIndex, endIndex)
Arguments
- text – Required. The text string containing substring.
- startIndex – Required. The starting position of the substring. Index starts at 0.
- endIndex – Optional. The ending position of the substring. If blank goes until the end of the string
Example
Extract the 5 digit purchase order number from the middle of the string
Slice('PO-12345-US', 3, 8)
Result
12345
Split Function
Purpose
Split a text string into an array of values by specifying a delimiter
Syntax
split(text, delimiter)
Arguments
- text – Required. The text string to be split into an array.
- delimiter – Required. The character to separate text strings upon
Example
Split the comma separated text string at each comma.
Split('01,3002,Accounts Receivable,50252.00', ',')
Result
[
'01',
'3002',
'Accounts Receivable',
'50252.00'
]
StartsWith Function
Purpose
Determine whether a substring is found at the beginning of a text string and return a true/false value
Syntax
startsWith(text1, text2, …)
Arguments
- text – Required. Text string where the substring may be located
- searchText – Required. Substring to look for at the start of the text string
Example
Check if the file name starts with “Timesheet”.
startsWith('Timesheet_MD_20231124.xlsx', 'Timesheet')
Result
true
Substring Function
Purpose
Extracts a substring given number of characters from the middle of a supplied text string
Syntax
substring(text, startIndex, length)
Arguments
- text – Required. The text string to extract a substring from.
- startIndex – Required. The starting position of the substring. Index starts at 0.
- length – Optional. The length of the substring. If blank goes until the end of the string.
Example
Extract the 5 digit purchase order number from the middle of the string
substring('PO-12345-US', 3, 5)
Result
12345
ToLower Function
Purpose
Convert a text string to lowercase
Syntax
toLower(text)
Arguments
- text – Required. The text string to convert to lowercase.
Example
Convert the email address to all lowercase characters
toLower('[email protected]')
Result
[email protected]
ToUpper Function
Purpose
Convert a text string to uppercase
Syntax
toUpper(text)
Arguments
- text – Required. The text string to convert to uppercase.
Example
Convert the month of January to Uppercase
toUpper('January')
Result
JANUARY
Trim Function
Purpose
Removes blank spaces from the start and the end of a text string
Syntax
trim(text)
Arguments
- text – Required. The text string with blank spaces at the start or end
Example
Remove the blank spaces from the start and end of the email address
trim(' [email protected] ')
Result
[email protected]
Did You Enjoy This Article? 😺
Subscribe to get new Power Apps articles sent to your inbox each week for FREE
Questions?
If you have any questions or feedback about All Power Automate Text Functions (With Examples) please leave a message in the comments section below. You can post using your email address and are not required to create an account to join the discussion.
Hey Matthew. Slight typo on your startswith function
Jon,
Fixed. I appreciate you sending the comment 🙂
Great article there are a few things I thought would be beneficial to add and noticed a few typos:
I enjoy reading your articles and look forward to trying out some of these text functions I haven’t used before.
Rhonda,
Many thanks for your comments. Here’s my changelog.
1. Added the word length.
2. Here’s how the GUID parameter letters work:
D: 5e097653-8071-4427-beb7-5287db3e535d
B: {5e097653-8071-4427-beb7-5287db3e535d} {with squiggly brackets}
N: 5e09765380714427beb75287db3e535d (no dashes)
P: (5e097653-8071-4427-beb7-5287db3e535d) (with rounded brackets)
X: A strange set of hexadecimal values that I do not understand
3. Updated from isFloat to isInt.
4. Testing and changed result to 7.
5. Retested my expression Slice(‘O-12345-US’, 3, 8) and it appears 12345 is returned correctly.
6. As mentioned, addressed in a prior comment… thanks!
7. Updated toLower to trim function.
If you retested with this expression Slice(‘O-12345-US’, 3, 8) it would return 12345 as it is missing the P from the original expression. Slice(‘PO-12345-US’, 3, 8), however I am not a power automate user yet and could be wrong, but just wanted to confirm, since your expression wasn’t exact as the example and I read that it didn’t include the last digit value.
Also update language on nthIndex Example
Find the final comma in the comma-separated text string
Rhonda,
Upon re-testing, the 2nd parameter appears to be zero-indexed. Therefore:
Slice(‘O-12345-US’, 3, 8) would return 2345-
Slice(‘PO-12345-US’, 3, 8) would return 12345
Thanks for the clarification.
Great article Matthew
This is great Matthew. I assume your favourite text function is the conCAT function.
Several appear to be copy/paste issues. Like endsWith(text1, text2, …).
Thank you so much for this. It is a gift!
Eileen,
Glad you are enjoying this.
correct me if I am wrong, NthIndexOf Function
you are looking for the 2nd comma not the final comma?
Ibraheem,
Thanks for the note, I’ve updated it 🙂
Amazing summary! It would be great if you could also describe some specific business or technical scenarios where these functions could be particularly useful.
Damian,
I’ve tried to make all of the examples something makers would do in the real world.
Any idea why this site lists Left, Right, and other String functions that do not appear to actually be in Power Automate, despite the page being a Microsoft page of Power Automate String functions.
Makes for confusion. Are these planned functions that just have not been released yet? Or a copy/paste error on the part of Microsoft, and they inadvertently posted the Power Apps String functions?
https://learn.microsoft.com/en-us/power-automate/minit/string-operations
Thoughts?
Hi Matthew, how to convert value to string? Examplae date to string, or int to string.
Thanks
Iwan,
Use either the formatNumber or formatDate function.
Great article. Would love to see an example of how to find a word after a string match. i.e. find “First Name” and match the word after it.