How To Validate A Power Apps Form Before Submission
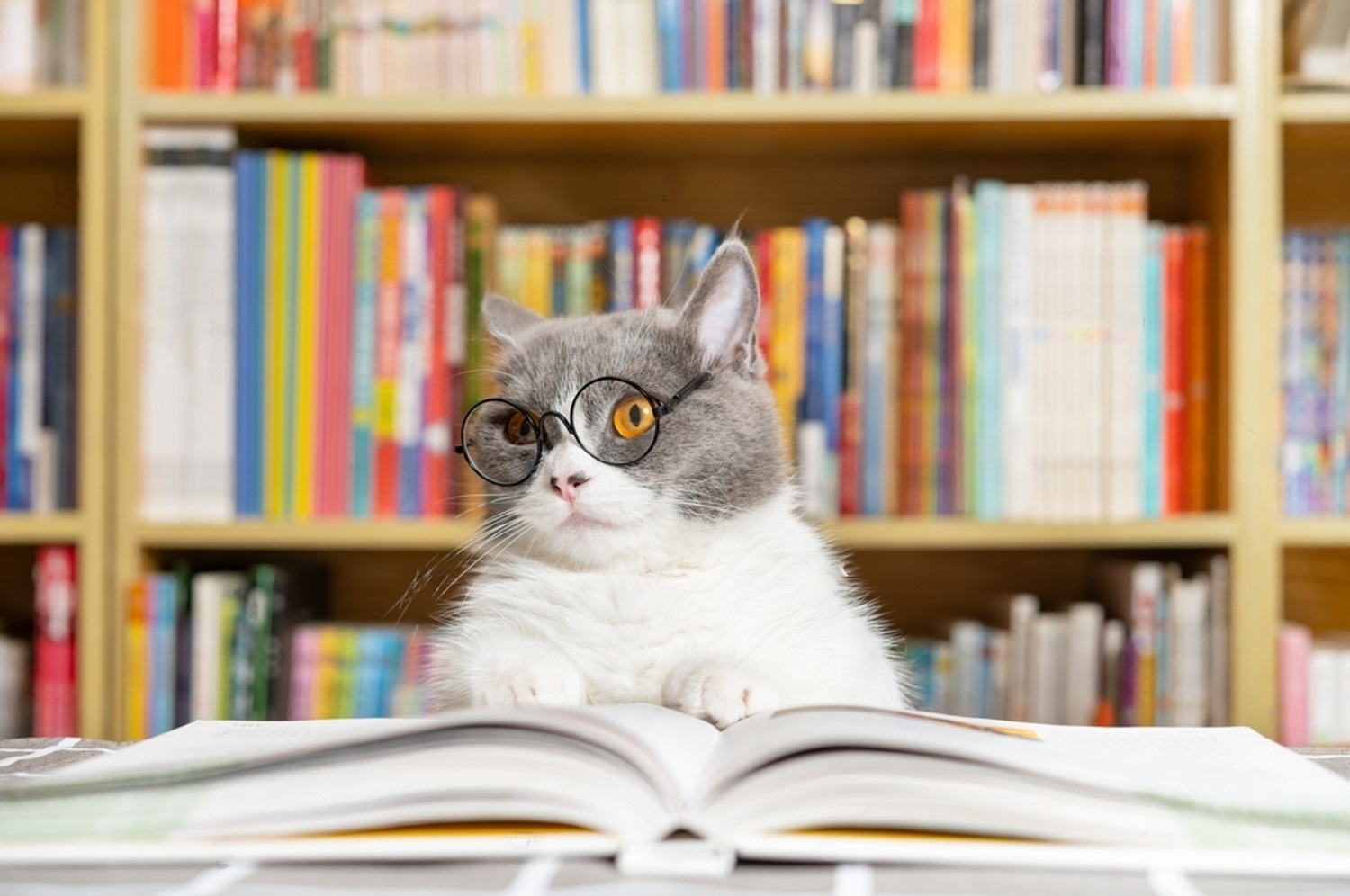
You can validate a Power Apps form before submission to ensure data is not saved until all criteria are met. For example, a text input might be required to have a value. Dates may need to be entered within a specific range. Or a phone number might have to be in a specific format. If data validation is not passed for any field, it should appear in an error state and show an helpful error message describing how to fix the issue.
Table of Contents
• Introduction: The Reserve A Room App
• Setup The SharePoint List
• Create A Power Apps Form
• Validate A Required Text Input Field
• Validate A Number In A Text Input Field
• Validate A Date Picker Field
• Validate A Phone Number In A Text Input Field
• Validate An Email In A Text Input Field
• Submit The Form If Data Validation Is Passed
• Test The Form Validation Rules
Introduction: The Reserve A Room App
Employees at a real estate firm use the Reserve A Room app to book a room for client meetings. The employee fills-in a form to book a room.
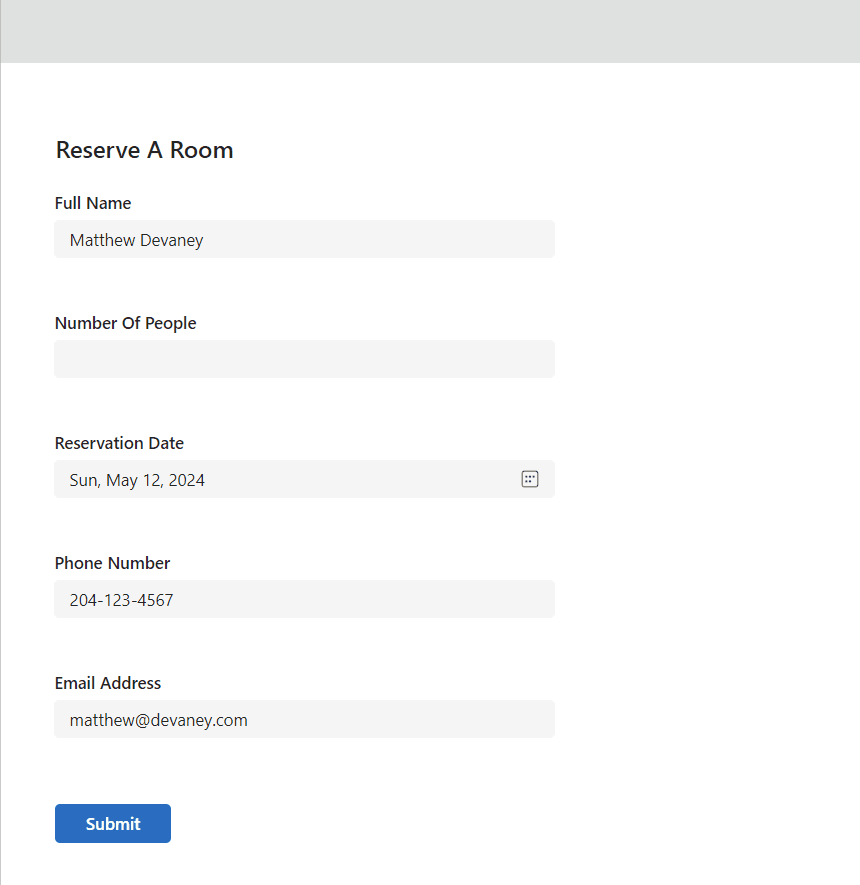
The rules are used to validate the Power Apps form. If an input field does not pass data validation the field turns red and an error message appears below.
- Full name is required
- Number of people attending must be greater than 0
- Reservation date cannot be in the past
- Phone number must be valid
- Email address must be valid
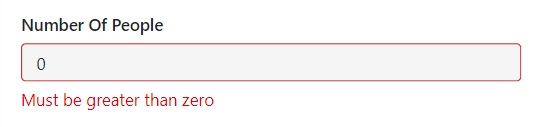
Setup The SharePoint List
Create a SharePoint list with the following fields and data types. Do not populate the list with any data.
- FullName (text)
- NumberOfPeople (number)
- ReservationDate (date)
- PhoneNumber (text)
- EmailAddress (text)
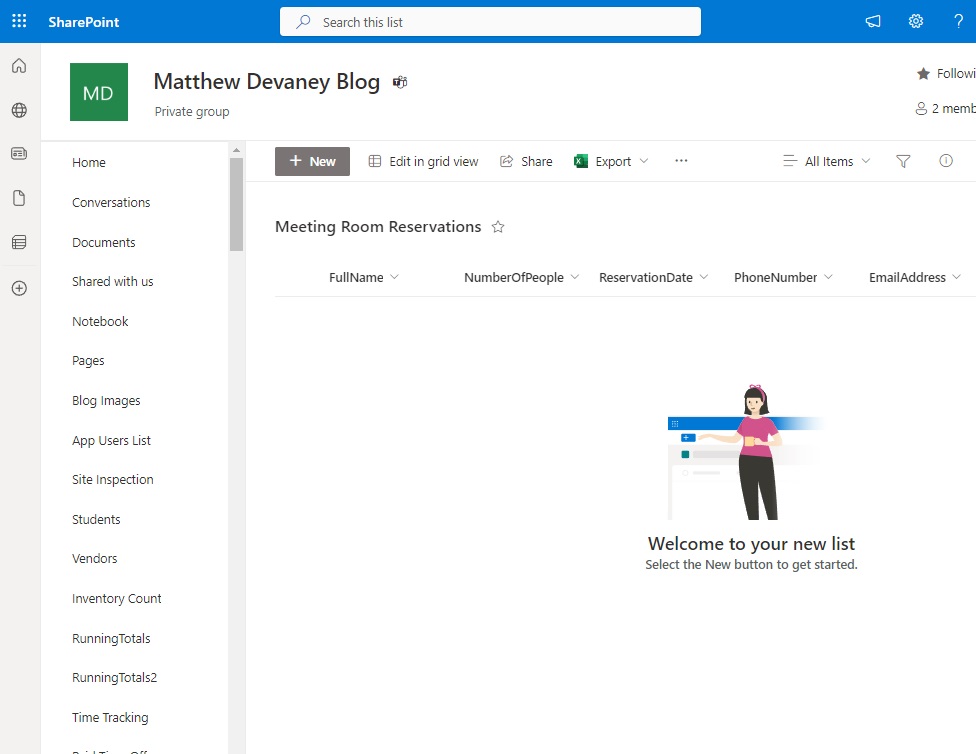
Create A Power Apps Form
Open make.powerapps.com and start a new app from blank. Connect the app to the Meeting Room Reservations SharePoint list. Then insert a form control onto the screen.
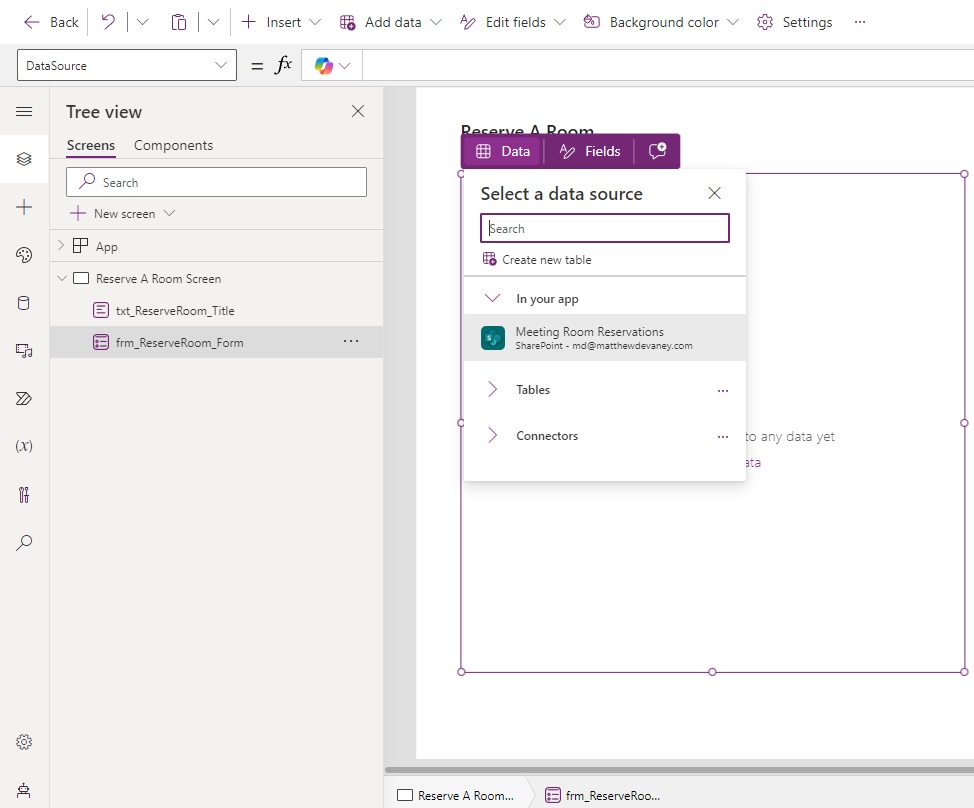
Use this code in the Items property of the form.
'Meeting Room Reservations'
Arrange the form fields in the following order shown below.
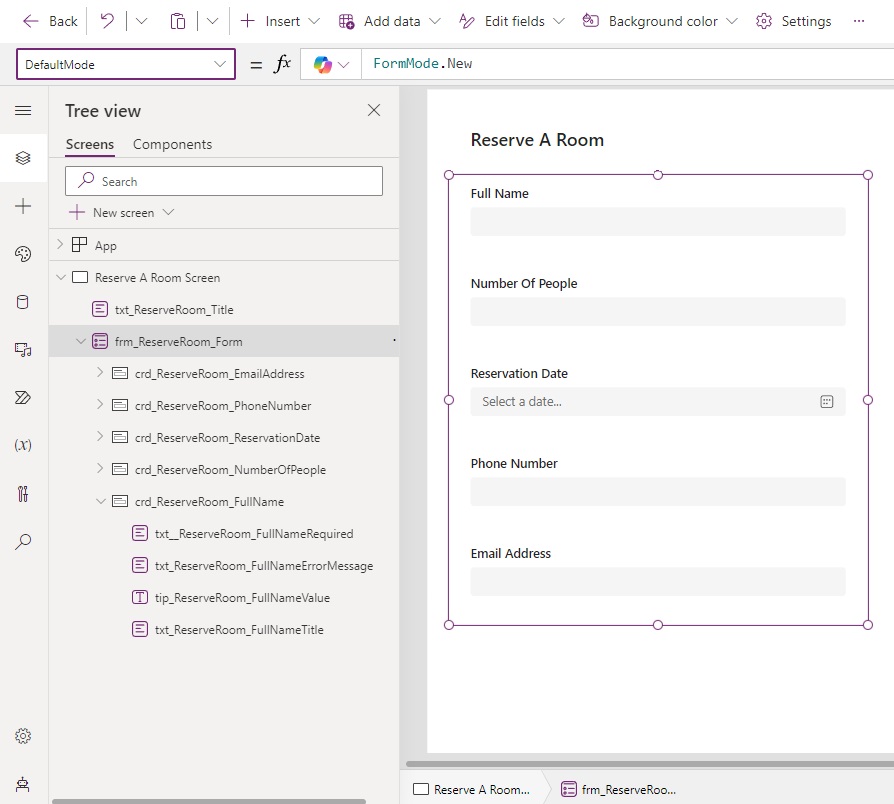
Then set the FormMode property of the form to this code. When the form is submitted it will add new values to the datasource.
FormMode.New
Validate A Required Text Input Field
The full name field of the form is a required field. It must be populated before the form can be submitted.
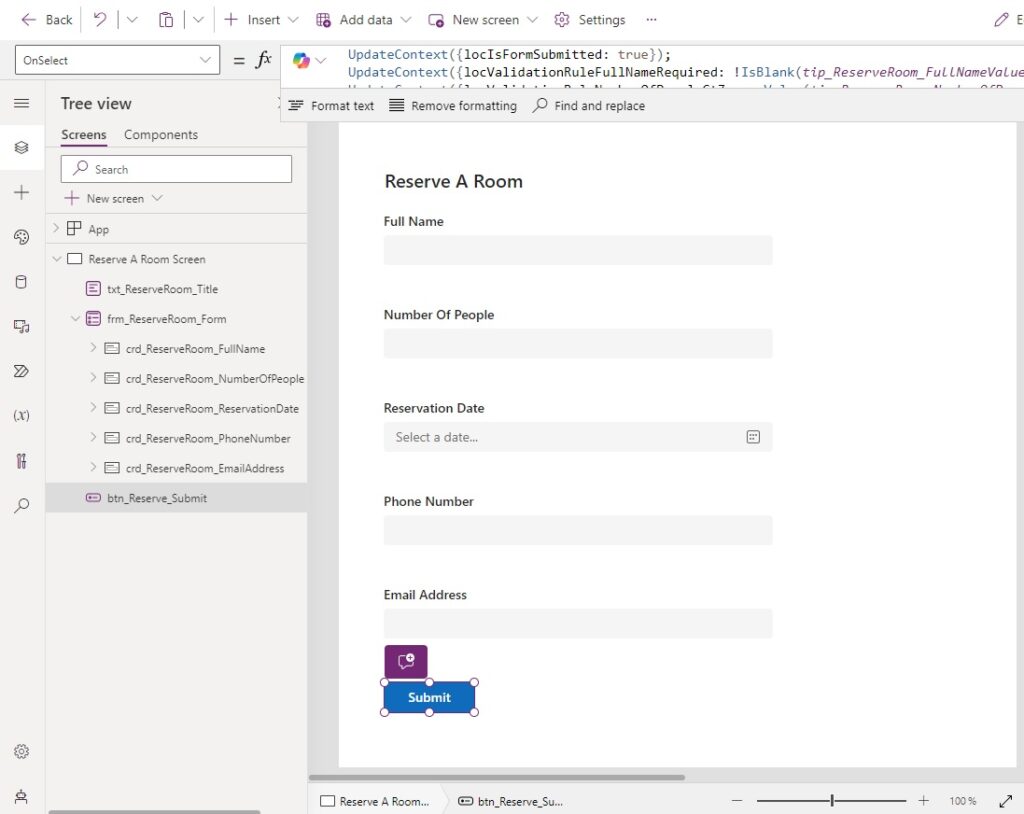
Add a button below the form with the text Submit. Then add this code to the OnSelect property of the button. The variable locIsFormSubmitted tracks whether the form has been submitted. The other variable locValidationRuleFullNameRequired checks if the Full Name text input has a value. If it does have a value the variable is set to true. Otherwise, it will remain false.
UpdateContext({locIsFormSubmitted: true});
UpdateContext({locValidationRuleFullNameRequired: !IsBlank(tip_ReserveRoom_FullNameValue.Value)});
If the data validation rule for Full Name is not passed the text input should have a red border.
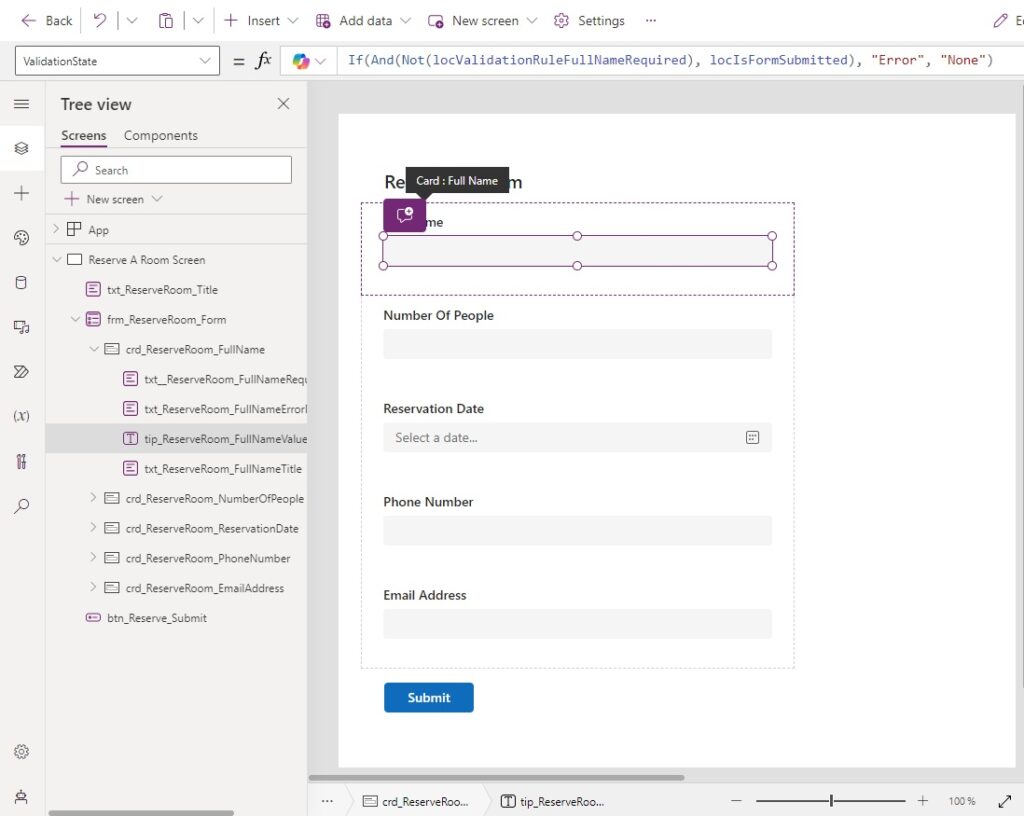
Set the ValidationState property of the text input to this code.
If(And(Not(locValidationRuleFullNameRequired), locIsFormSubmitted), "Error", "None")
An error message should also appear below the Full Name text input instructing the user on how to correct the error.
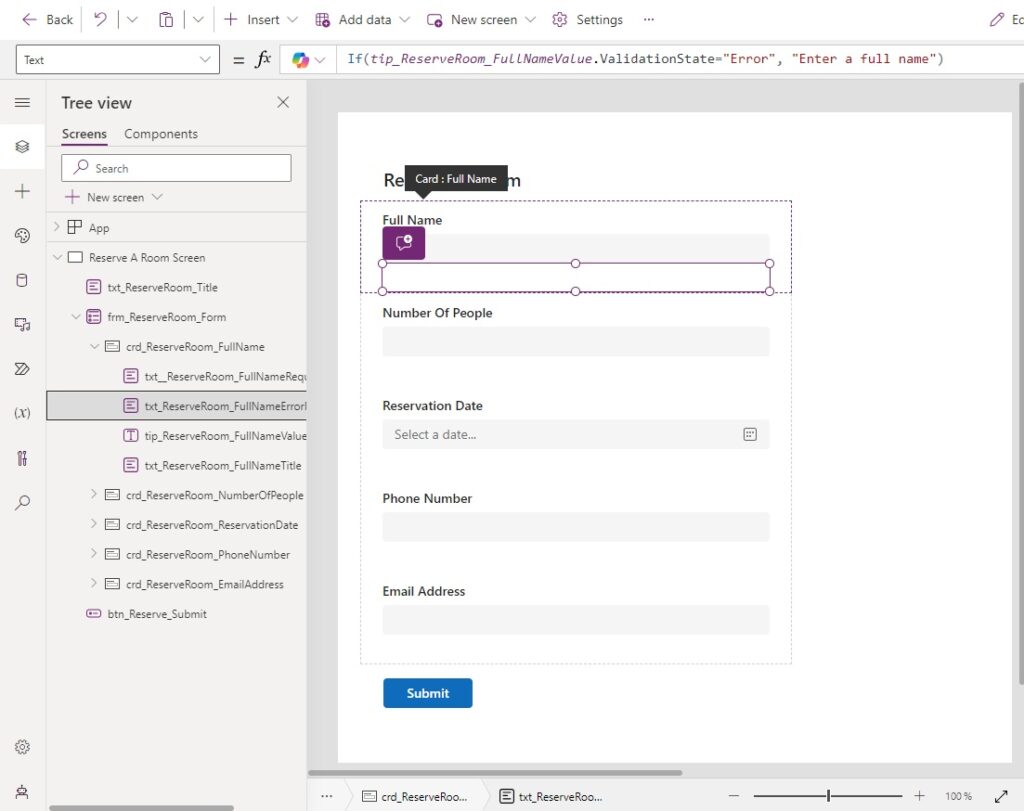
Write this code in the Value property of the text control below the text input. It will show an error message when data validation is not passed.
If(tip_ReserveRoom_FullNameValue.ValidationState="Error", "Enter a full name")
Set the FontColor property of the text input to red.
Color.Red
When the form is submitted and the full name field has no value it will look like this:
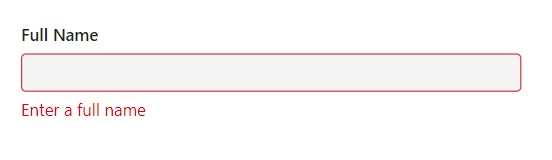
Validate A Number In A Text Input Field
The number of people using the meeting room must be greater than zero. Otherwise the form should not submit.
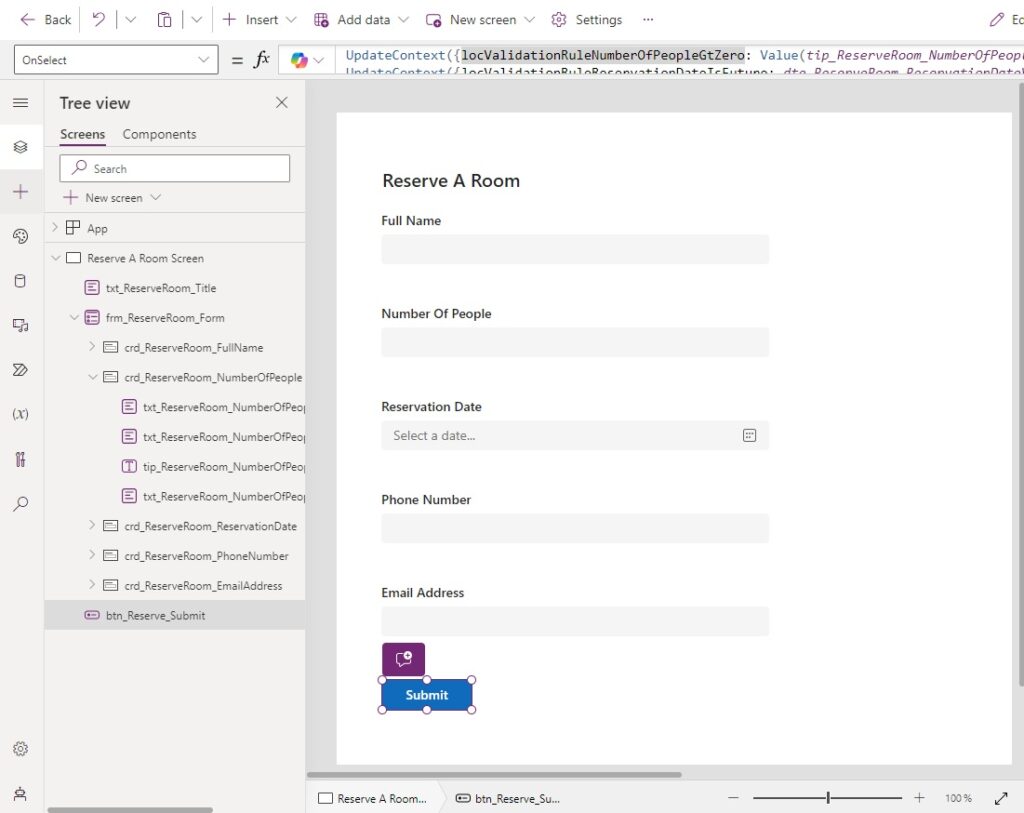
Add this code to the OnSelect property of the submit button to check if the value input is greater than zero.
UpdateContext({locValidationRuleNumberOfPeopleGtZero: Value(tip_ReserveRoom_NumberOfPeopleValue.Value) > 0});
When the data validation rule is not passed for the Number Of People field its border should turn red.
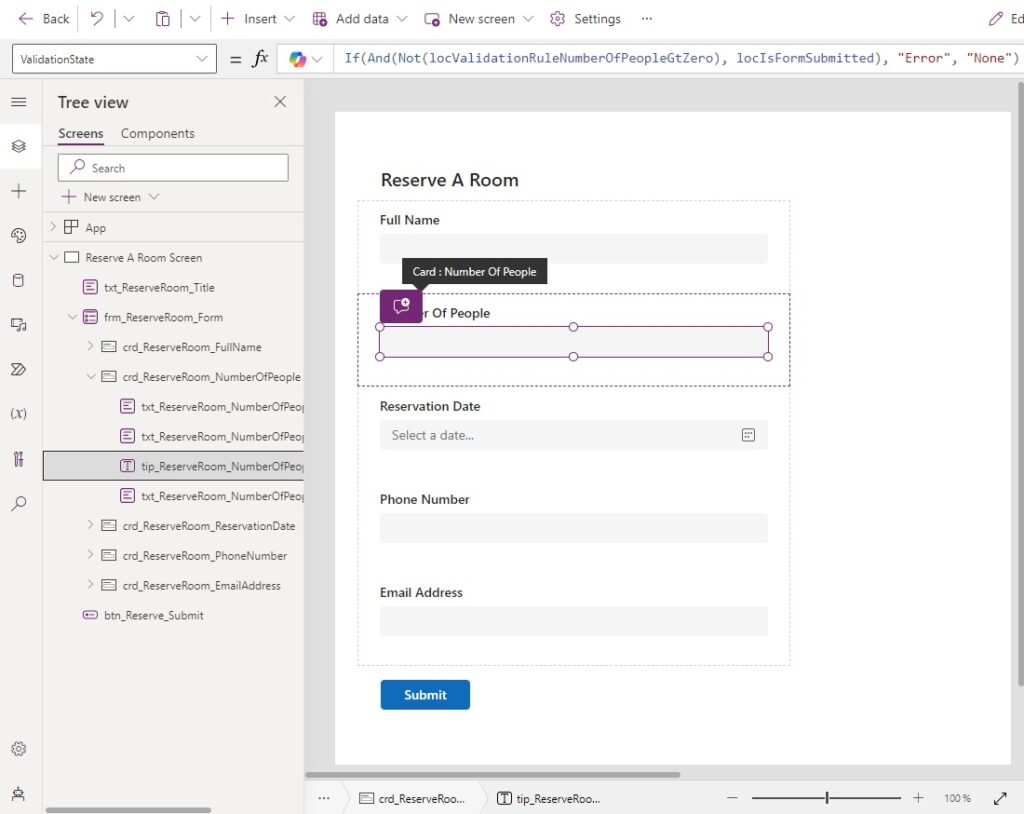
Use this code in the ValidationState property of the Number Of People text input to show an error.
If(And(Not(locValidationRuleNumberOfPeopleGtZero), locIsFormSubmitted), "Error", "None")
An error message appears when the Number Of People field is in an error state.
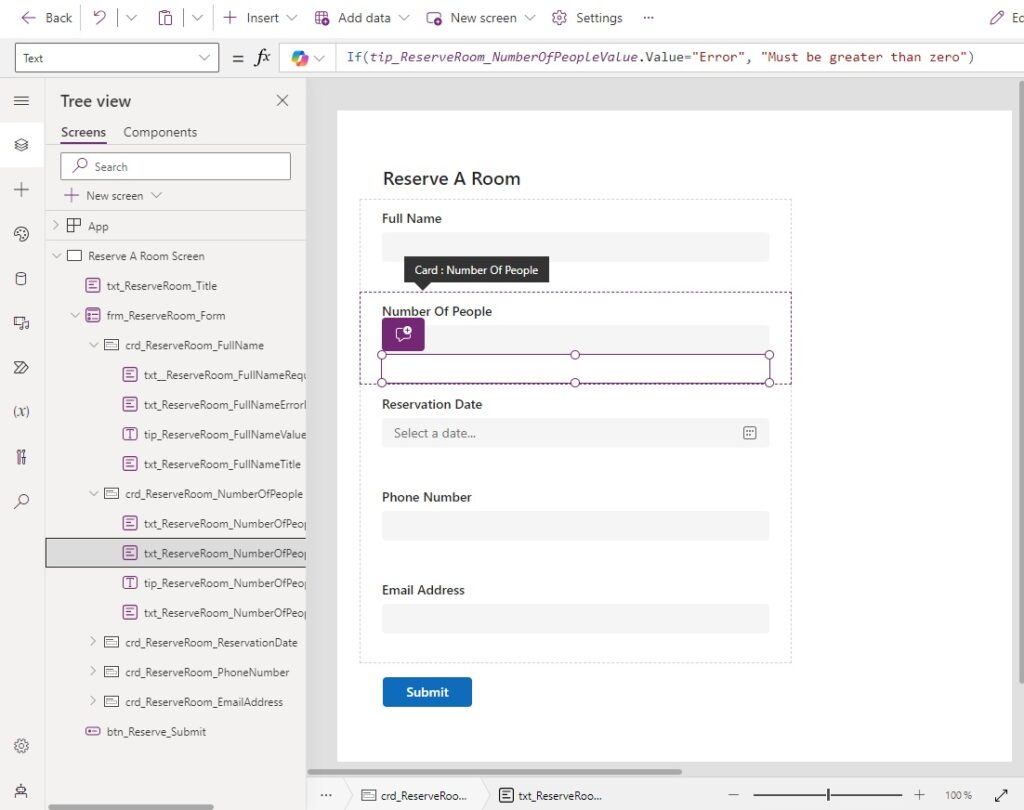
Use this code in the Value property of the text control to show an error message.
If(tip_ReserveRoom_NumberOfPeopleValue.ValidationState="Error", "Must be greater than zero")
And set the FontColor property of the text input to red.
Color.Red
When data validation is not passed for the Number Of People field it appears like this.
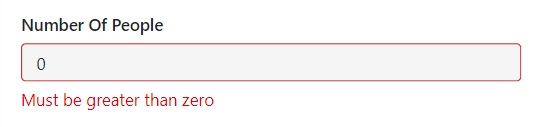
Validate A Date Picker Field
A valid reservation date must be no more than 90 days in the future. When this criteria is not met the form cannot be submitted.
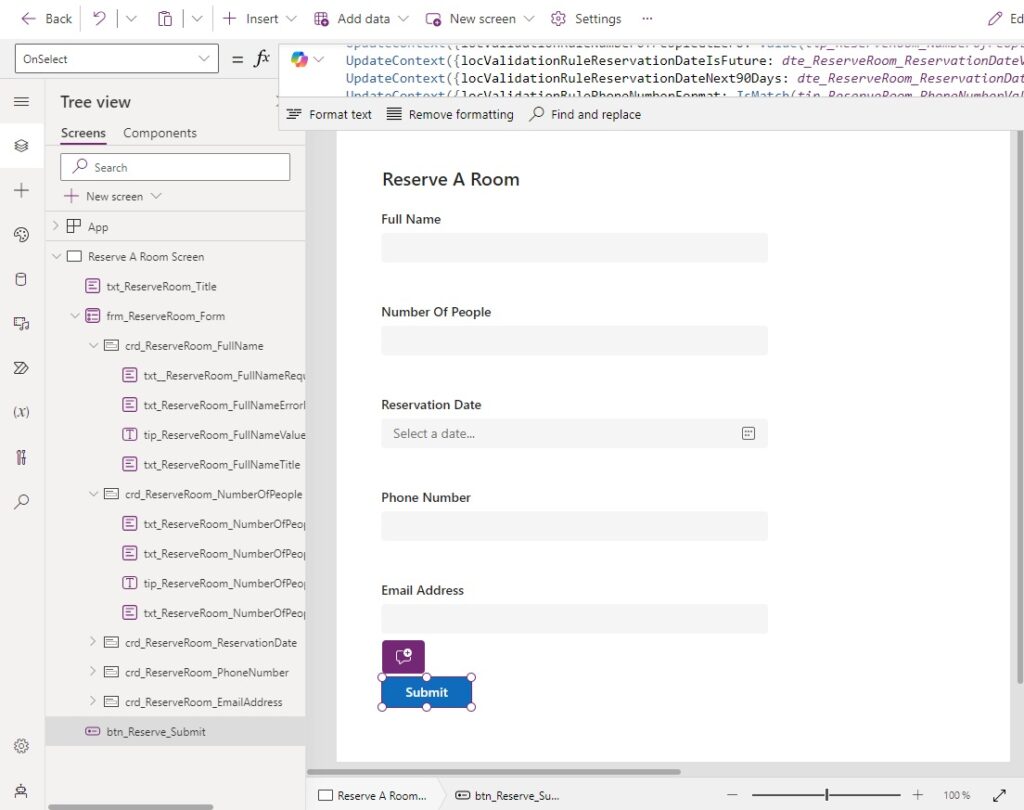
Add this code to the OnSelect property of the submit button. The first variable locValidationRuleReservationDateIsFuture checks to see if the date is in the future. And the second variable locValidationRuleReservationDateNext90Days determines if the date is less than 90 days away.
UpdateContext({locValidationRuleReservationDateIsFuture: dte_ReserveRoom_ReservationDateValue.SelectedDate > Today()});
UpdateContext({locValidationRuleReservationDateNext90Days: dte_ReserveRoom_ReservationDateValue.SelectedDate-Today() <= 90});
The reservation date field will be shown in an error state if both data validation rules are not passed.
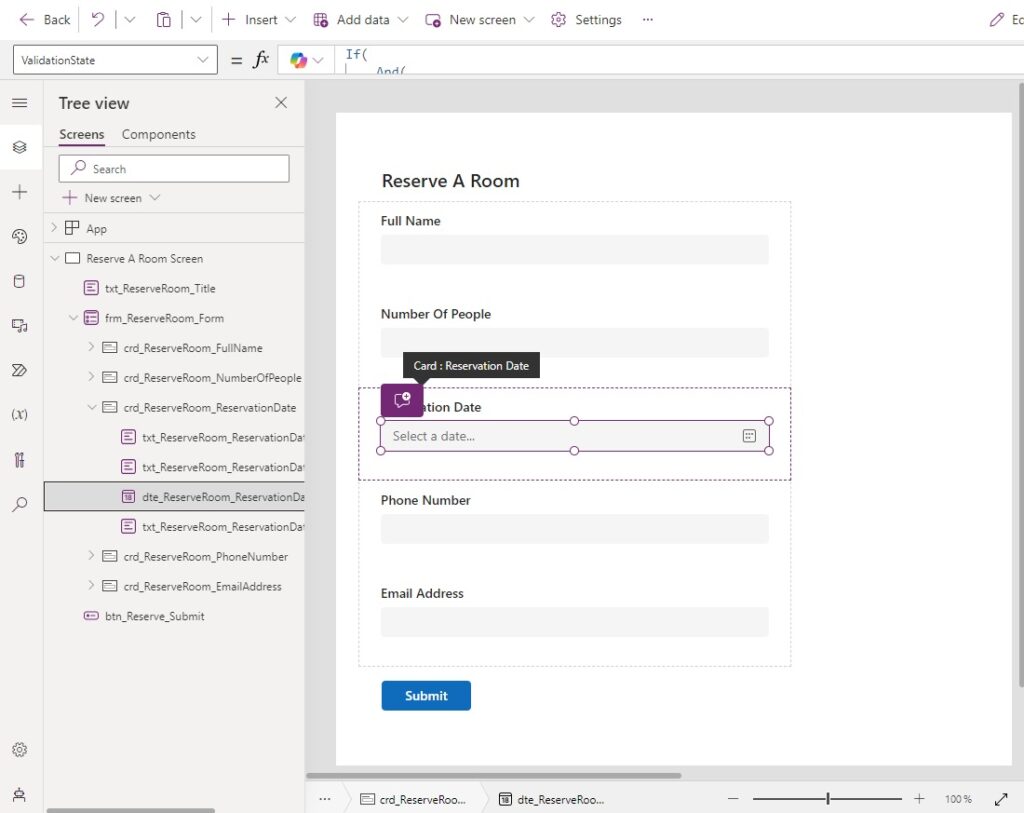
Use this code in the ValidationState property of the date picker.
If(
And(
Or(
Not(locValidationRuleReservationDateIsFuture),
Not(locValidationRuleReservationDateNext90Days)
),
locIsFormSubmitted
),
"Error",
"None"
)
A different error message will appear depending on which date picker validation rule is not passed.
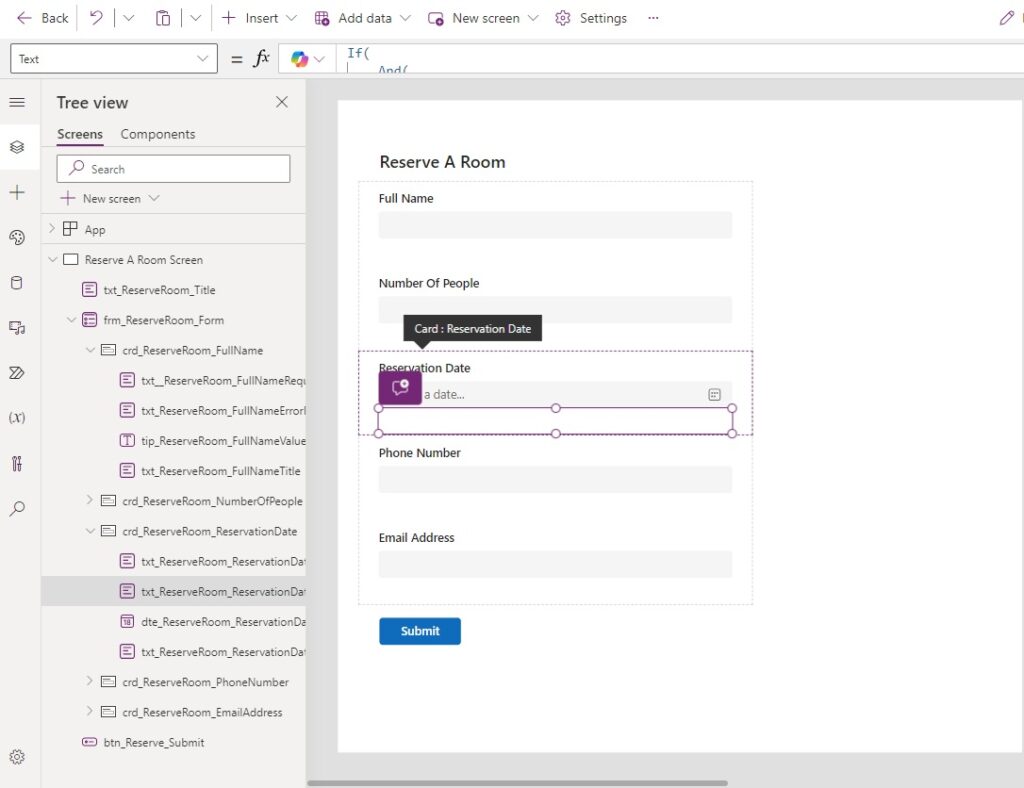
Write this code in the Value property of the error message label. When the date entered is today or in the past the message “Must be a future date” shows. Or if the date entered is more than 90 days away the message “Must be within 90 days” appears.
If(
And(
locValidationRuleReservationDateIsFuture,
dte_ReserveRoom_ReservationDateValue.ValidationState = "Error"
),
"Must be a future date",
And(
locValidationRuleReservationDateNext90Days,
dte_ReserveRoom_ReservationDateValue.ValidationState = "Error"
),
"Must be within the next 90 days"
)
Change the FontColor property of the error message to red.
Color.Red
The possible error states for the date picker looks like these images. Please note, the date picker does not have a red border due to a bug with the modern controls.
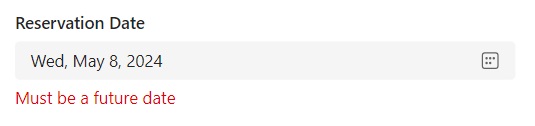
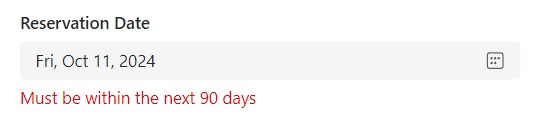
Validate A Phone Number In A Text Input Field
A phone number must be entered in the specific format ###-###-####. If not, the form will show an error upon submission.
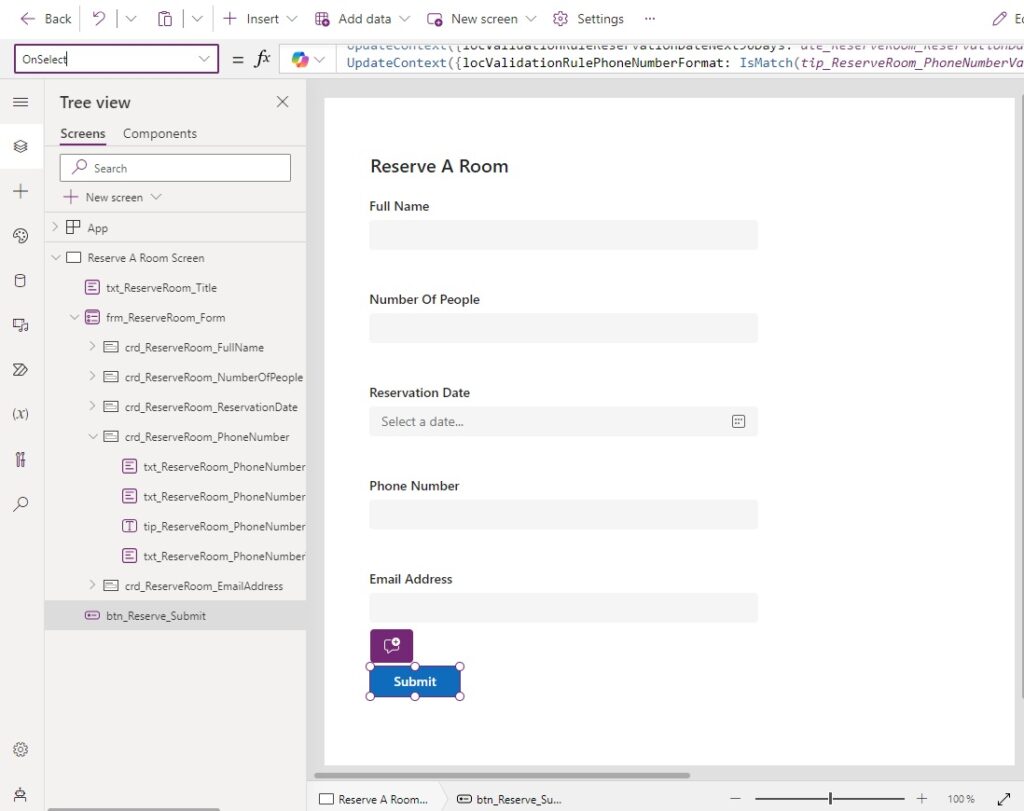
Add this code to the OnSelect property of the submit button. It uses the IsMatch function to check if the phone number entered matches a regular expression.
UpdateContext({locValidationRulePhoneNumberFormat: IsMatch(tip_ReserveRoom_PhoneNumberValue.Value, Match.Digit&Match.Digit&Match.Digit&"-"&Match.Digit&Match.Digit&Match.Digit&"-"&Match.Digit&Match.Digit&Match.Digit&Match.Digit)});
When the phone number does not match the expected format the text input should appear in an error state.
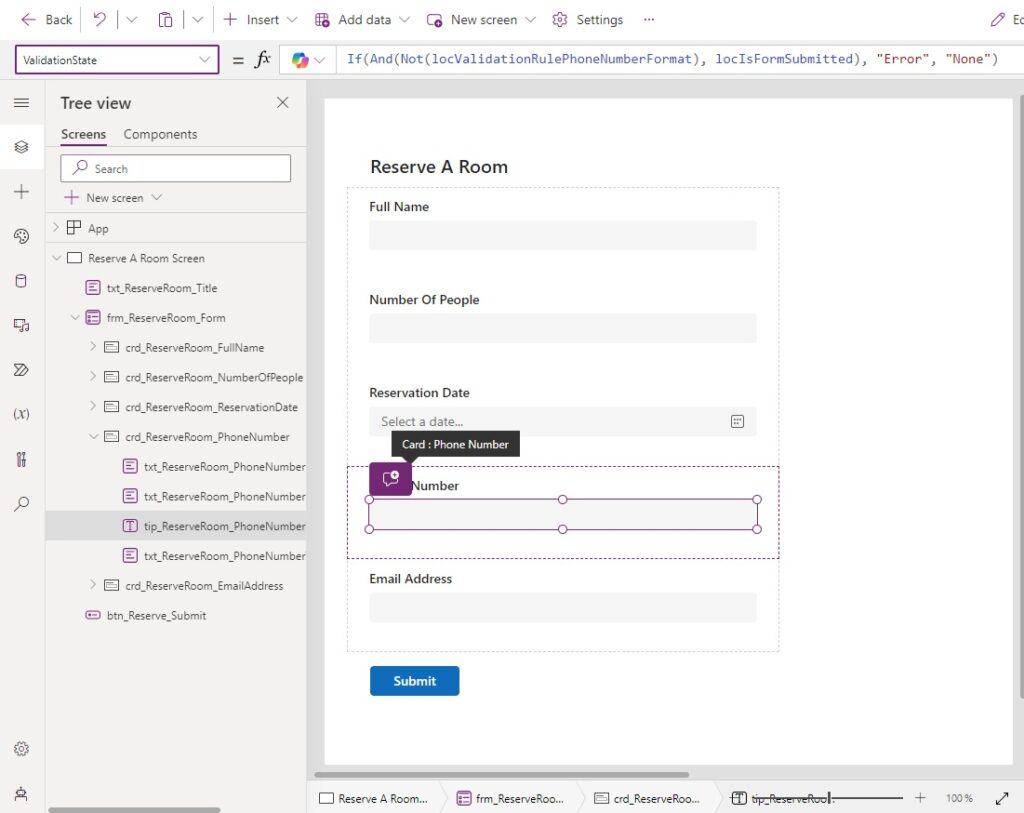
Write this code in the ValidationState property of the text input.
If(And(Not(locValidationRulePhoneNumberFormat), locIsFormSubmitted), "Error", "None")
An error message should appear when data validation for the phone number format is not passed.
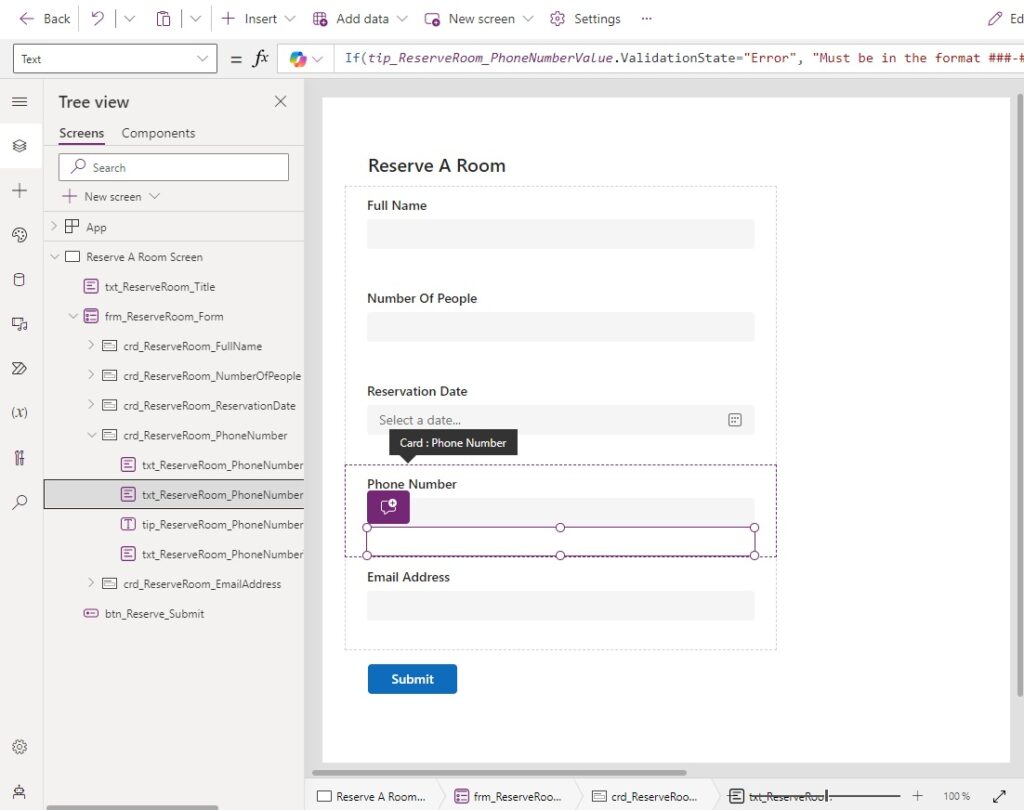
Write this code in the Value property of the text field to display the error message when the phone number text input is in an error state.
If(tip_ReserveRoom_PhoneNumberValue.ValidationState="Error", "Must be in the format ###-###-####")
The phone number field looks like this while in an error state.
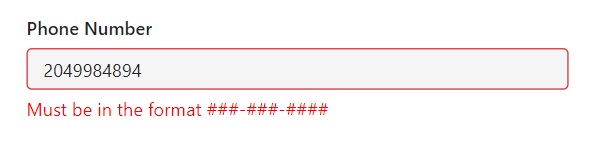
Validate An Email In A Text Input Field
The email address field requires the user to input a valid email. Otherwise the form will not submit.
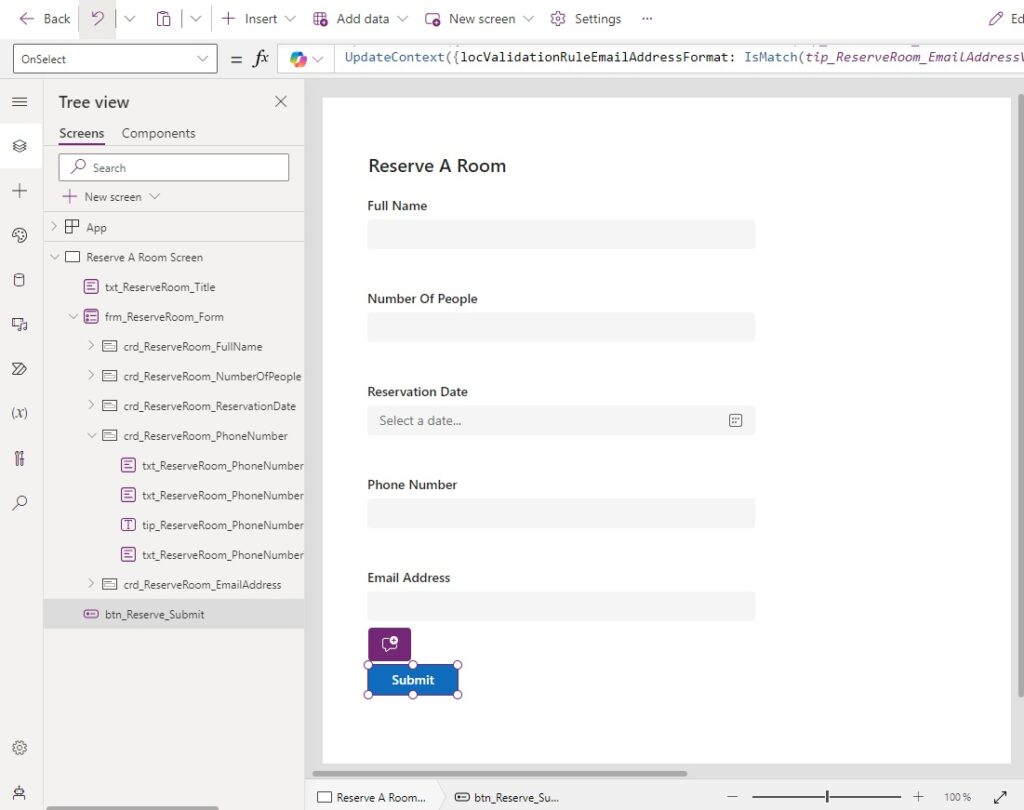
Add this code to the OnSelect property of the submit button. The IsMatch function uses a pre-generated regex string called Match.Email to determine if the email is valid.
UpdateContext({locValidationRuleEmailAddressFormat: IsMatch(tip_ReserveRoom_EmailAddressValue.Value, Match.Email)});
The email address field will show an error when data validation is not passed.
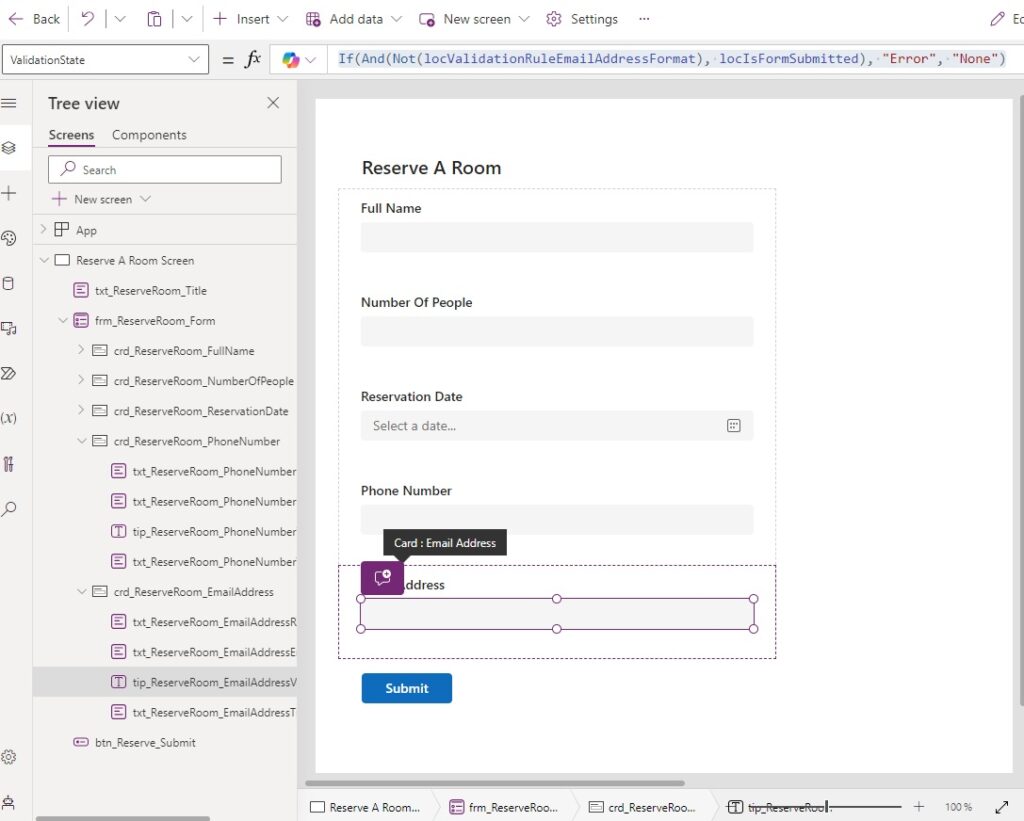
Write this code in the ValidationState property of the email address text input.
If(And(Not(locValidationRuleEmailAddressFormat), locIsFormSubmitted), "Error", "None")
A helpful error message showing the correct phone number format will be shown when the text input is in an error state.
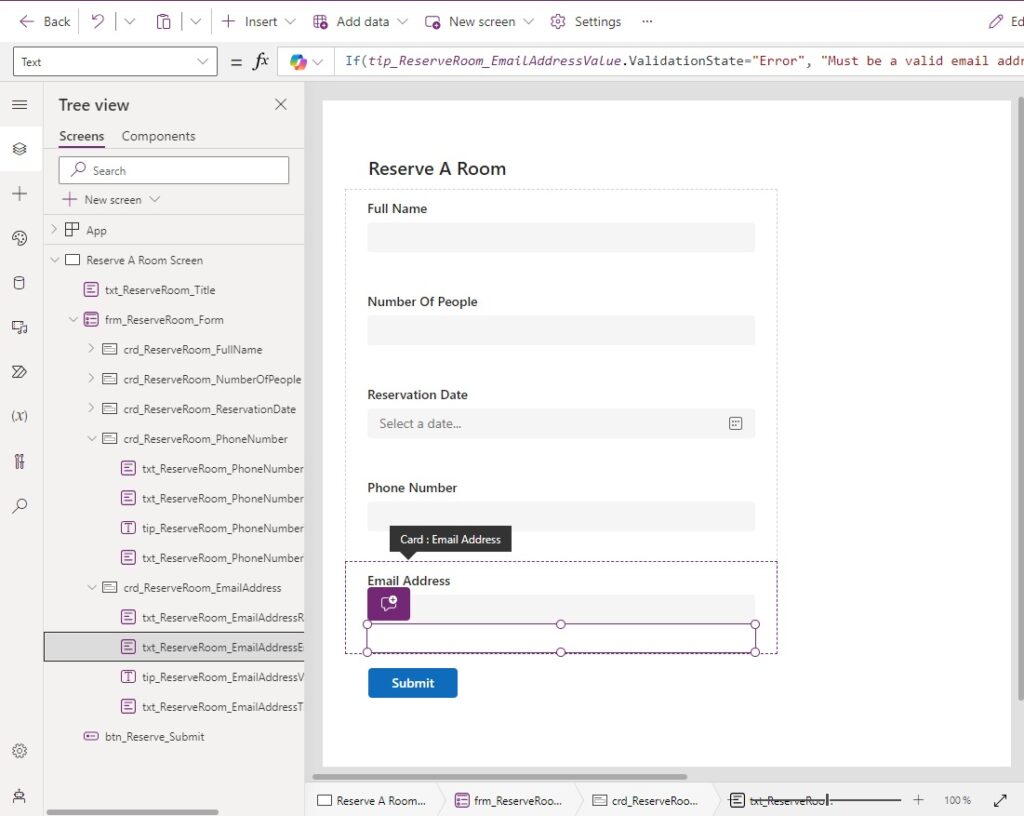
Write this code in the Value property of the phone number text input to display an error message.
If(tip_ReserveRoom_EmailAddressValue.ValidationState="Error", "Must be a valid email address")
When the email address text input is in an error state it looks like this.
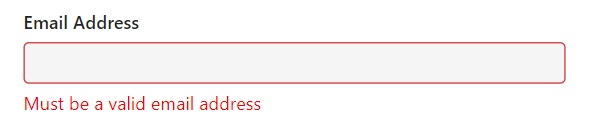
Submit The Form If Data Validation Is Passed
The form should only be submitted if all data validation rules are passed.
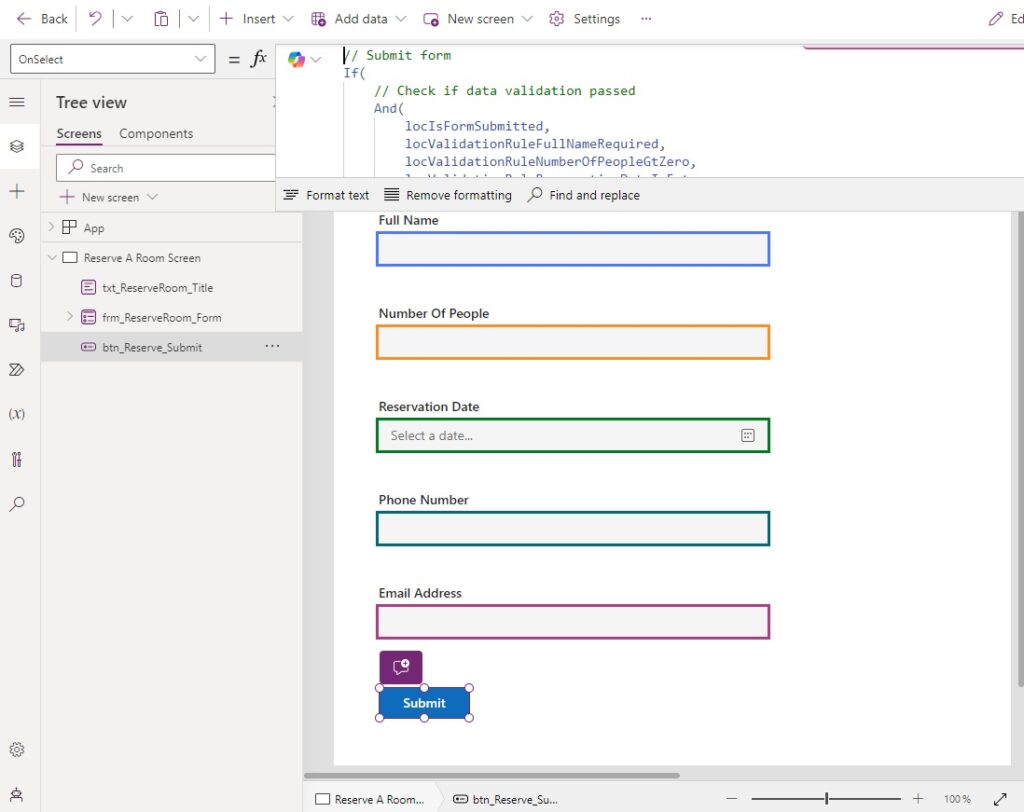
Write this code in the OnSelect property of the submit button. It checks several data validation rules to see if they passed or failed. Then if we successfully validate the Power Apps form it is submitted.
The validation rules will already be present in the code from our previous actions. We only need to add the code below the submit form comment.
// Validation Rules
UpdateContext({locIsFormSubmitted: true});
UpdateContext({locValidationRuleFullNameRequired: !IsBlank(tip_ReserveRoom_FullNameValue.Value)});
UpdateContext({locValidationRuleNumberOfPeopleGtZero: Value(tip_ReserveRoom_NumberOfPeopleValue.Value) > 0});
UpdateContext({locValidationRuleReservationDateIsFuture: dte_ReserveRoom_ReservationDateValue.SelectedDate > Today()});
UpdateContext({locValidationRuleReservationDateNext90Days: dte_ReserveRoom_ReservationDateValue.SelectedDate-Today() <= 90});
UpdateContext({locValidationRulePhoneNumberFormat: IsMatch(tip_ReserveRoom_PhoneNumberValue.Value, Match.Digit&Match.Digit&Match.Digit&"-"&Match.Digit&Match.Digit&Match.Digit&"-"&Match.Digit&Match.Digit&Match.Digit&Match.Digit)});
UpdateContext({locValidationRuleEmailAddressFormat: IsMatch(tip_ReserveRoom_EmailAddressValue.Value, Match.Email)});
// Submit form
If(
// Check if data validation passed
And(
locIsFormSubmitted,
locValidationRuleFullNameRequired,
locValidationRuleNumberOfPeopleGtZero,
locValidationRuleReservationDateIsFuture,
locValidationRuleReservationDateNext90Days,
locValidationRulePhoneNumberFormat,
locValidationRuleEmailAddressFormat
),
// Data validation passed
SubmitForm(frm_ReserveRoom_Form),
// Data validation failed
Notify(
"Please correct all errors before submitting the form",
NotificationType.Error
)
)
Once the form is submitted the user should see a success notification. Use this code in the OnSuccess property of the form to show the notification and capture the record last submitted record.
Notify(
"Meeting room reservation was made successfully",
NotificationType.Success
);
Set(gblReserveARoom, frm_ReserveRoom_Form.LastSubmit);
Then write this code in the Item property of the form to indicate which record to display when the form is in new mode or edit mode.
gblReserveARoom
Test The Form Validation Rules
We are now finished writing the code to validate a Power Apps form. Save and publish the app then test it in play mode to ensure it is working as expected.
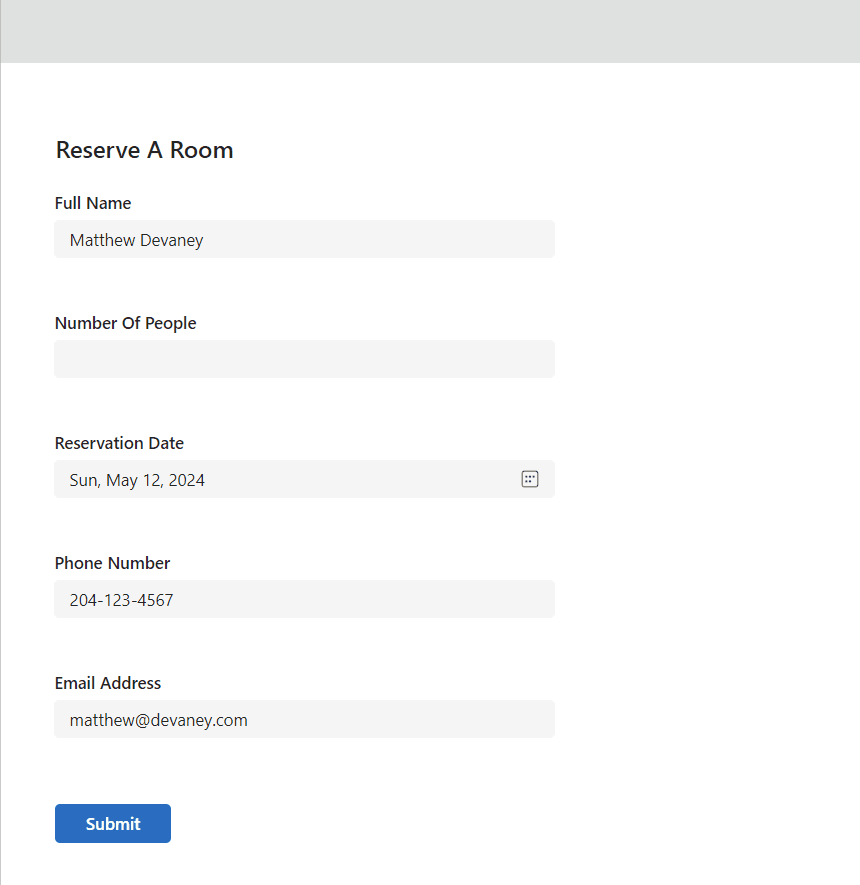
Did You Enjoy This Article? 😺
Subscribe to get new Power Apps & Power Automate articles sent to your inbox each week for FREE
Questions?
If you have any questions or feedback about How To Validate A Power Apps Form Before Submission please leave a message in the comments section below. You can post using your email address and are not required to create an account to join the discussion.
If only everyone explained power apps in this simple method. I have a similar app, but only use two rooms which they book out for the entire day.
If I wanted to add an additional drop down with room 1, room 2 how would I validate it that it would only show the room available for that date, to prevent double booking? I assume I could use isblank if they don’t pick an entry to validate the second part.
James,
I would recommend placing a filter in the dropdown’s Items property. Here’s some sample code that I’ve not tested, but it will get you started.
Assume there are two SharePoint lists: ‘Meeting Rooms’ and ‘Meeting Room Reservations.’ The ‘Meeting Room Reservations’ table has a lookup column to the room named MeetingRoomField.
Filter(AddColumns(‘Meeting Rooms’, IsRoomAvailable, LookUp(‘Meeting Room Reservations’, And(MeetingRoomField.ID=ThisRecord.ID, ReservationDate=ReservationDate.SelectedDate))=Blank()), IsRoomAvailable = true)
Great post!
One side question is do you think it’s safe to use these modern controls for any new solutions since now.
Good question! I am wondering the same.
Wei,
I think we are very close. And its tempting to use them because there will be a technical debt later on for any apps not using those controls.
I am using a modern form. When setting datacards required property to true. Only one field at a time validates and combo box doesn’t validate at all. I will try using your method for the form validation including combo box.
I also have a patch form that I’ve made using containers and modern controls. To validate this I created a collection with properties for the field name, required (bool) and isValidated (bool). I am using OnChange to patch the isValidated property of that field. The submit button can then check which fields have been validated and show relevant user feedback.
If( IsBlank(gender.Selected.Value), Notify(“Please fill in all required fields.”), SubmitForm(Form_edit) ) /// the Gender is a combo box /// hope it helps
Can there be an instance, where a user has selected the value from a dropdown and submitted and the value patched to SP list is blank?
Udula,
Yes, it could be blank if the PATCH code is not properly configured.
Thank you, it is an interesting article and easy to get, i like the idea of using contextual variables with And Function
I don’t have a property called ValidationState under the text field. Does anyone know why?
Thanks
Sam,
You must use the Power Apps modern controls set which includes the ValidationState property. Classic controls do not have it.
Great, I found it now.
Thank you! 😊
great Post, thank you.
I wonder if it would be better to add this code as well in the onchange property of the inputs field for a more instant feedback if there are several errors on the form:
what do you think?
Micha,
Yes, this is called “live validation” and I’ve done it in many Power Apps. I would encourage you to do it too.
Hey Matt
on the new date picker now it has a property StartDate (long time due this one)
this gray out all anything before selected date
just loving it…..
this way you can avoid all the validation for futurte date once the you add somethiung like
thanks for all your posts
I enjoy your blog posts as they are simple to consume and always have great insights. From a UX standpoint, I’d not enable the Submit button until all the required fields are entered. Also, would give the user a fair caveat that all fields are mandatory unless otherwise indicated. Since, the validation code is on the submit button in your example, how would you recommend we change the validation code to cover the scenario where the button is enabled only after all the fields are populated?
Bhaskar,
I disagree with your UX comment. The Submit button should remain enabled at all times. A disabled button will confuse users and might make them believe the form cannot be submitted at all. The best solution is to give error messages after a failed data validation.
In the OnSelect property of the submit button, why create all the context variables? Could you just check that the validation state of each of the controls is none?
Mark,
I decided on using context variables so that the validation would not trigger immediately while the user is still typing in the box.
I have a Power App in which users have to answer a Yes or No question. If the user answers Yes, additional questions populate. These additional questions need to be answered before the user submits the form. I am struggling with validation for those additional questions. When I try to add validation for the additional questions, users receive an error for not completing all required questions if they answer No and the additional questions do not populate. Any guidance/help/suggestion would be greatly appreciated.
Check if the Yes/No question equals “true” in your validation logic. Something along the lines: UpdateContext({locValidationRuleFullNameRequired: tglAdditionalQuestionsNeeded.Value && !IsBlank(tip_ReserveRoom_FullNameValue.Value)});
That way the validation rule will be true only if the Yes/No question is “Yes” and the additional field is blank.
This is a great post to describe validation with modern controls! Thank you!
One question I have is how can we seamlessly integrate this with any existing validation the data source might already build in to the app, for example a field that is required in SharePoint?
Matthew, have you been noticing an issue with Powerapps? I have a form in edit mode (connected to a SharePoint list), and a button with only SubmitForm(formname). I’m seeing today it’s taking two clicks of this button, or two calls to SubmitForm(formname) in order to post the update to the SharePoint list.
It ‘looks’ like it’s successful with one call to SubmitForm(formname). Even the OnSuccess event is triggered. But no update.
Help!
Hi Matthew,
How to populate LastSubmit data in NewForm?
Eventually, how to get a value of field for LastSubmit and populate in appropriate field in NewForm. I’m using SharePoint integration.
I thought to use DefaultSelectedItems.
Any advice
Thanks in advance,
pyx